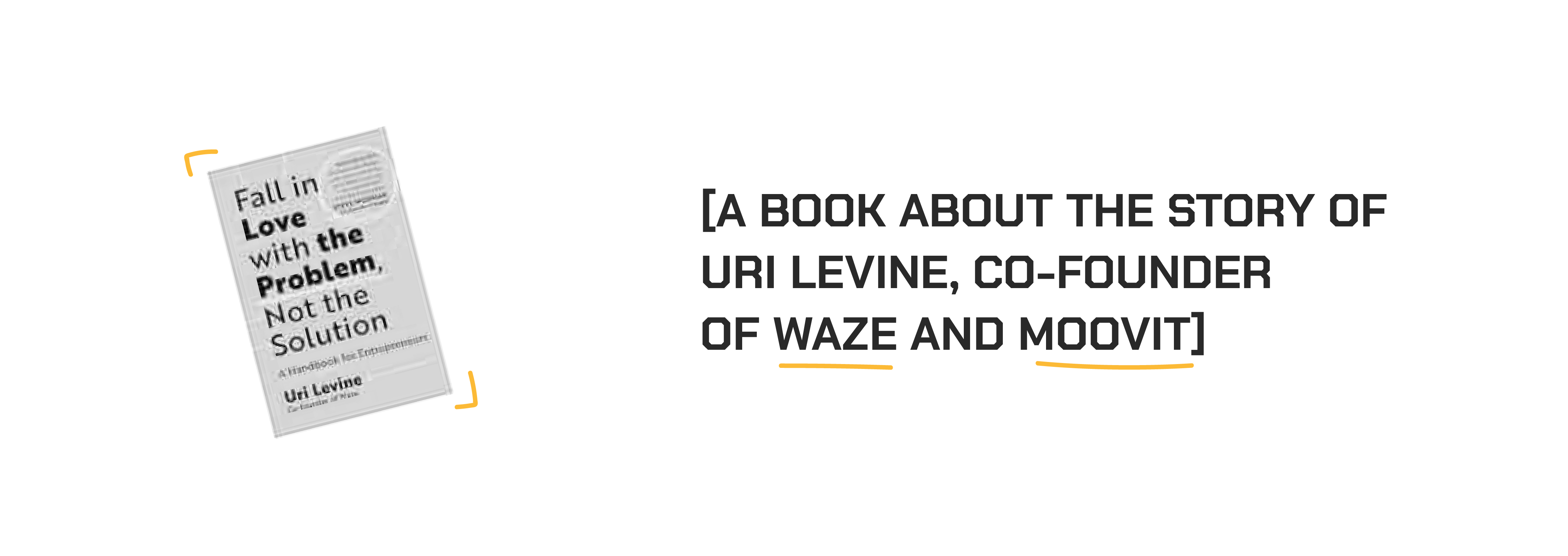
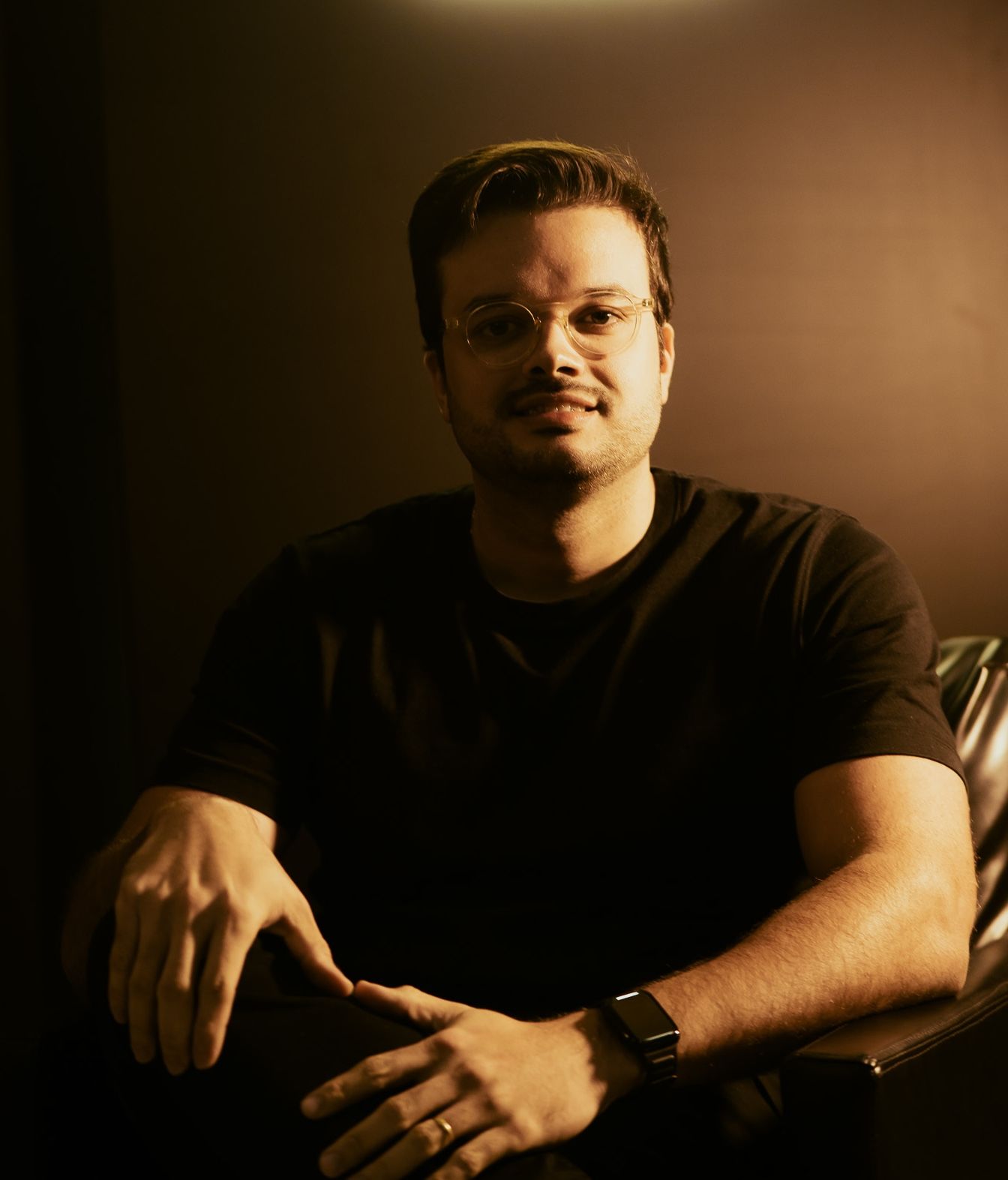
João Cardoso
23 de agosto de 2024
Developing efficient backend servers is crucial for companies that want to offer fast and reliable services. In this context, the Go language, or Golang, has gained prominence as a popular choice among developers looking for performance, simplicity and scalability.
Go was created by Google to combine the efficiency of languages like C with the simplicity of languages like Python. Since then, Go has become an excellent choice for building backend servers, thanks to its unique features.
Go offers native support for concurrent programming with goroutines, which are lightweight threads managed by the language. With goroutines, it's possible to perform several tasks at the same time efficiently, which is essential for backend servers that have to deal with many simultaneous requests.
Goroutine example
package main
import (
“fmt”
“time”
)
func process(id int) {
fmt.Printf(“Processing task %d\n”, id)
time.Sleep(2 * time.Second)
fmt.Printf(“Task %d completed\n”, id)
}
func main() {
for i := 1; i <= 5; i++ {
go process(i)
}
time.Sleep(3 * time.Second)
fmt.Println(“All tasks completed”)
}
With goroutines, you can perform simultaneous tasks simply and efficiently, maximizing the use of server resources.
Simplicity is one of the pillars of Go. The language is designed to be easy to learn and use, with a clear and straightforward syntax. This allows developers to write efficient and maintainable code, reducing development time and the occurrence of bugs.
Example of an http server in Go
package main
import (
"fmt"
"net/http"
)
func helloHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, world!")
}
func main() {
http.HandleFunc("/", helloHandler)
http.ListenAndServe(":8080", nil)
}
In just a few lines, you can create a working HTTP server. The simplicity of the syntax makes the code easy to understand and modify.
Go is a compiled language, which means that the code is transformed into performance-optimized binaries before being executed. This, combined with its efficient memory management, allows Go servers to be fast and use fewer resources, which is vital for applications that need to scale quickly
HTTP Request Handling Example
package main
import (
“fmt”
“log”
“net/http”
“sync”
)
var counter int
var mu sync.Mutex
func counterHandler(w http.ResponseWriter, r *http.Request) {
mu.Lock()
counter++
fmt.Fprintf(w, “Visits: %d\n”, counter)
mu.Unlock()
}
func main() {
http.HandleFunc(“/counter”, counterHandler)
log.Fatal(http.ListenAndServe(“:8080”, nil))
}
With this example, you can see how Go efficiently handles concurrent operations, ensuring that the server remains fast and responsive.
Go offers a number of robust tools that facilitate development, such as the module system for package management, an integrated linter (golint) and code formatting tools (gofmt). In addition, the rich collection of standard libraries and the active community make development in Go even more efficient.
Large companies such as Google, Uber and Twitch have adopted Go for their backend servers due to its efficiency and ability to handle large volumes of traffic. These examples highlight Go's ability to operate on a large scale, providing a fast and reliable user experience.
Go offers a unique combination of simplicity, performance and robustness, making it ideal for backend server development. Whether for startups building an MVP or large companies optimizing their infrastructure, Go provides the tools needed to achieve these goals efficiently.
If you haven't yet explored Go for backend development, this is the perfect time to start. With a smooth learning curve and a growing ecosystem, Go could be the key to taking your web development to the next level.
Get in touch to ask questions or request our services.